This Article describes how to install Apache Tomcat 7 on a Debian (Lenny) OS in 8 easy steps.
For this HowTo to work smoothly you must already have Java installed on your machine. In case you haven’t Java installed on your machine, there is anoter HowTo for this Task on my Blog. Follow the instructions in this Post and come back when Java is up and running.
1. Download it!
The first step is to aquire Tomcat 7 by downloading it from the Homepage. This is really easy, I used wget to download the “apache-tomcat-7.0.2.tar.gz” archive into my /temp Directory. This should look something like this:
cd /tmp
wget http://apache.prosite.de/tomcat/tomcat-7/v7.0.2-beta/bin/apache-tomcat-7.0.2.tar.gz
2. Unzip & Move
Move the package to it’s permanant location and unzip the package into its own folder.
mv apache-tomcat-7.0.2/ /usr/local/tomcat
tar -zxvf apache-tomcat-7.0.0.tar.gz
3. Create “tomcat” Group & User
Next you need to add a new group and a new user to your system. This will be the user and the group under which the Tomcat server runs.
1. To add a group called “tomcat” you simply type:
groupadd tomcat
2. Now you have to create a new user called “tomcat” (useradd tomcat) who belongs to the group “tomcat” (-g tomcat). You also should set the home directory of that user to the directory where you moved the Tomcat server in the previous step. In this case that would be “/usr/local/tomcat” (-d /usr/local/tomcat). So you should end up with a statement that looks something like this:
useradd -g tomcat -d /usr/local/tomcat tomcat
3. Now you should also add the user to the “www-data” group. This group should already exist. You do that by executing the following command:
usermod -G www-data tomcat
4. Create INIT File for Tomcat
Now you should create an INIT-File that makes it possible to start, stop and restart your Tomcat Server. This file must be located in your “/etc/init.d/” directory. You can use the following command to create a file called “tomcat” and open up that file in an editor (I used nano).
nano /etc/init.d/tomcat
Now you should add the following lines into the file an save it:
#Tomcat auto-start
#description: Auto-starts tomcat
#processname: tomcat
#pidfile: /var/run/tomcat.pid
#this path should point to your JAVA_HOME Directory
export JAVA_HOME=/usr/lib/jvm/java-6-sun
case $1 in
start)
sh /usr/local/tomcat/bin/startup.sh
;;
stop)
sh /usr/local/tomcat/bin/shutdown.sh
;;
restart)
sh /usr/local/tomcat/bin/shutdown.sh
sh /usr/local/tomcat/bin/startup.sh
;;
esac
exit 0
Make sure you set the right paths for the startup.sh and shutdown.sh scripts. They reside in the /bin directory of your tomcat path (use the path to which you moved the tomcat files in step 2).
5. Adjust Permissions of INIT File
Since you have to execute the tomcat file, you have to assign the correct rights for the file to be executable.
This line should do the trick:
chmod 755 /etc/init.d/tomcat
6. Make Tomcat auto-start on boot (optional)
If you want the Tomcat Server to start every time the system boots up you can use the “update-rc.d” command to set a symbolic link at the correct runlevel. For the “tomcat fle” this looks like this:
update-rc.d tomcat defaults
Now the Tomcat Server starts automatically at system bootup. This step is optional you can always start your Tomcat Server manually like this:
/etc/init.d/tomcat start
7. Modify Tomcat Users File
We are almost there! In this step we need to add a user in the tomcat-users.xml. This user is used to gain access to the Tomcat Manager Interface in the next step. So open up the “tomcat-users.xml” file with any editor you like (i used nano):
nano /usr/local/tomcat/conf/tomcat-users.xml
There is a <tomcat-users> section within that file. After the installation this section should only contain comments and look something like this:
<tomcat-users>
<!--
NOTE: By default, no user is included in the "manager-gui" role required
to operate the "/manager/html" web application. If you wish to use this app,
you must define such a user - the username and password are arbitrary.
-->
<!--
NOTE: The sample user and role entries below are wrapped in a comment
and thus are ignored when reading this file. Do not forget to remove
<!.. ..> that surrounds them.
-->
<!--
<role rolename="tomcat"/>
<role rolename="role1"/>
<user username="tomcat" password="tomcat" roles="tomcat"/>
<user username="both" password="tomcat" roles="tomcat,role1"/>
<user username="role1" password="tomcat" roles="role1"/>
-->
</tomcat-users>
Now all we need to do is add a new user by adding some new lines here. After insertion the section should look like this:
<tomcat-users>
<!--
NOTE: By default, no user is included in the "manager-gui" role required
to operate the "/manager/html" web application. If you wish to use this app,
you must define such a user - the username and password are arbitrary.
-->
<!--
NOTE: The sample user and role entries below are wrapped in a comment
and thus are ignored when reading this file. Do not forget to remove
<!.. ..> that surrounds them.
-->
<!--
<role rolename="tomcat"/>
<role rolename="role1"/>
<user username="tomcat" password="tomcat" roles="tomcat"/>
<user username="both" password="tomcat" roles="tomcat,role1"/>
<user username="role1" password="tomcat" roles="role1"/>
-->
<role rolename="manager"/>
<role rolename="manager-gui"/>
<role rolename="admin"/>
<user username="admin" password="tomcat" roles="admin,manager,manager-gui"/>
</tomcat-users>
In lines 19-22 I added three roles with the names “manager”, “manager-gui” and “admin”. In line 22 I created a user with the username “admin”, the password “tomcat” and the roles I created before. This user will be used to access the Tomcat Manager Interface in the next step.
All there is left to do is to restart the Tomcat Server to make him recognize that the “tomcat-users.xml” file has changed and that there is a new user with the name “admin” and the password “tomcat”. This is how you restart your Tomcat Server:
/etc/init.d/tomcat restart
8. Test Tomcat Manager Interface
Finally we can check if everything went right. If your Tomcat Server runs on your local machine your can access it via the following adress:
http://localhost:8080/
otherwise you have to replace the “localhost” part with the IP adress or name of your server. This could look like this:
http://192.168.6.15:8080/
If all went right you should see the following site in your browser:

Tomcat Site
Now you know that your Tomcat Server is running. Next we will log in to the Tomcat Manager Interface. For that you should click on the link that says “Tomcat Manager” in the upper left part of the webpage (This link is actually highlighted in the picture above.). Now you will be prompted for your username and password. Type in the username and the password you set in the “tomcat-users.xml” file (in this case that would be “admin” and “tomcat”)
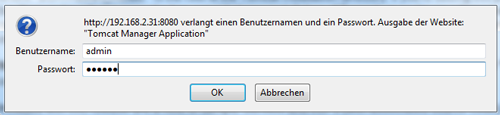
Tomcat Manager Prompt
After that you should see the site of the Tomcat Manager.
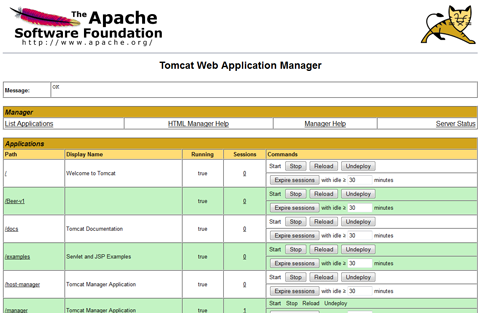
Tomcat Manager Interface
Congratulations you just installed your very own Tomcat Server.
Let me know what you think about my article. Perhaps there are some sections where i need to be a little bit more elaborate. Just drop me a line….